Documentation - Scripting
To get interaction in the 3D Model you can use the powerful scripting API. The API is inspired by Excel’s VBA. The language ist Javascript.
You need to understand basic concepts of programming and of javascript to use this powerful tool. You can do many things with the javascript API:
create interaction in the 3D-Model
shorten your Excel-Sheet data e.g. by using loops
do additional automation
The documentation for the scripting API is still in progress. There will be examples soon. Please write an E-mail if you need more information!
Don’t confound: The scripting API is not the developer API. The developer API is for additional GUI and heavier programming tasks whereas the Scripting API is used within sheetBuild’s Editor.
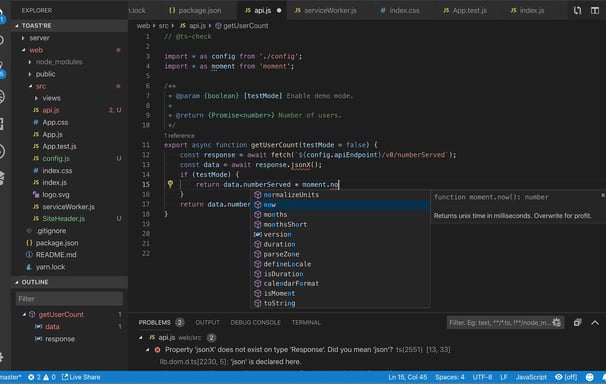
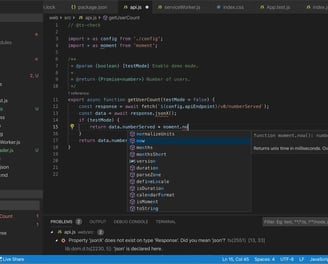